2.8. Focus and scroll management
You can set the focus to a clickable element (e.g., a
form input, link, or button) using the focus function of every DOM element. The
most helpful usage is for form input controls. The behavior varies on
different mobile browsers. On some touch devices, focusing in a text
box should automatically open the onscreen keyboard, and in some
cursor-based browsers it will position the cursor over the
element.
Note:
If the document the user is browsing is form-based, like a
search page or a contact us page, it will be better for usability to
automatically focus on the first text input. This reduces the amount
of navigation the user has to do on the page.
On some devices, the global window object has a scrollTo function that takes two parameters,
xPosition and yPosition, specifying the position at the
top-left corner of the screen to scroll to. On some devices (like the
iPhone), using scrollTo emulates
the user’s scrolling and hides the browser’s toolbars, as if the user
were scrolling with her fingers. So, for iPhone browsers, it is common
to use the following code, which automatically hides the toolbars
after the onload event:
window.scrollTo(0, 1);
This function can also be used to generate links to the top of
the page, on compatible devices:
<a href="javascript:window.scrollTo(0, 1);">Go to Top</a>
This same behavior can also be applied without JavaScript, using
anchors.
Table 10 lists the
different browsers’ compatibility with the focus and scrollTo functions.
Table 10. Focus and scrolling compatibility table
Browser/platform | focus | scrollTo |
---|
Safari | Yes | Yes |
Android
browser | Yes | Yes |
Symbian/S60 | Yes | Yes |
Nokia Series
40 | No | No |
webOS | No | No |
BlackBerry | No | No |
NetFront | No | Yes |
Internet
Explorer | No | Yes |
Motorola Internet
Browser | No | No |
Opera
Mobile | Yes | No |
Opera
Mini | No | No |
2.9. Timers
JavaScript offers two kinds of timers: setTimeout and setInterval. The first one is executed once
and the second one is executed every n
milliseconds until it is cancelled using clearInterval.
You can use timers for updating information from the server
using Ajax every n seconds, for creating an
animation, or for controlling the timeout of an operation.
Warning:
In mobile browsers, you need to be especially careful about
using timers because of the battery consumption. If you need to use many
high-frequency timers at the same time, try to manage them using
only one timer that will launch different behaviors from the same
process.
The first question we need to ask ourselves is, what happens
when our web page goes to the background because the user switches
focus to another application (in multitasking operating systems) or
opens or browses to another tab or window? Another problem is what
happens when the phone goes to sleep (because of the user’s inactivity
while the script is executing). The behavior of timers can be a little
tricky in these situations.
Yet another problem is that timers execute on the same thread as
the main script. If our script is taking too much processor time (a
normal situation with large scripts on low- and mid-end devices), our
timers will be delayed until some spare execution time is
found.
If we use a low frequency for the timer (for example, 10
milliseconds), the timer will generally have problems meeting the
timetable.
Remember that the JavaScript execution time depends a lot on the
device hardware and the browser’s engine. Even if they’re running the
same operating system, like Android, execution times can differ: for
example, an HTC G1 will be much slower than a Nexus One with a 1-Ghz
processor.
Let’s look at a simple example and see what happens normally and
when we send the web page to the background:
<!DOCTYPE html PUBLIC "-//WAPFORUM//DTD XHTML Mobile 1.0//EN"
"http://www.wapforum.org/DTD/xhtml-mobile10.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<title>Using Timers</title>
</head>
<body>
<script type="text/javascript">
var timer = setInterval(timerHit, 200);
var q = 0;
var lastTime = new Date().getTime();
function timerHit() {
q++;
var deltaTime = new Date().getTime() - lastTime;
document.getElementById("content").innerHTML += q + ": " +
deltaTime + "<br />";
lastTime = new Date().getTime();
// Generate some random delay
var randomNumber = Math.floor(Math.random()*1000)+5000;
for (var i=0; i<randomNumber; i++) {
var a = new Array();
}
// We will run only 15 experiments
if (q==15) {
clearInterval(timer);
}
}
</script>
<div id="content">
</div>
</body>
</html>
As shown in Figure 4, the real times
are very different on different devices. On low- and mid-end devices,
if they work at all, the result is far from our 200 ms intention—some
low-end devices don’t even accept timers with a frequency of less than
1 second.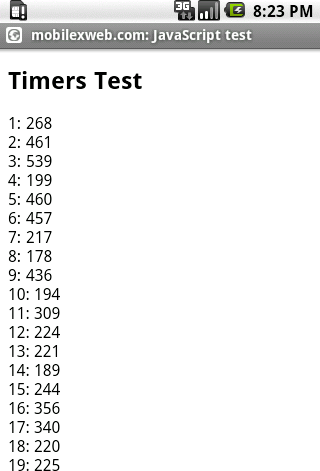
Table 11
shows which browsers support timers, and how they handle the timers
when the page is in the background.
Table 11. Timers support compatibility table
Browser/platform | Timers
available | Timers in
background |
---|
Safari | Yes | Stopped. From iOS 4.0:
continue working while in other browser's
window. |
Android
browser | Yes | Stopped. |
Symbian/S60 | Yes | Stopped. From 2.2:
continue working while in other browser's
window. |
Nokia Series
40 | No | |
webOS | Yes | Continue
working. |
BlackBerry | No | |
NetFront | Yes | No
multitasking. |
Internet
Explorer | Yes | Stopped. |
Motorola Internet
Browser | No | |
Opera
Mobile | Yes | Continue
working. |
Opera
Mini | No | |
Note:
The Gmail for Mobile team discovered some issues with timer
behavior on mobile Safari and Android devices, and made the results
public in the team blog at http://www.mobilexweb.com/go/timers. The conclusions
are: for low-frequency timers (1 second or more), there are no
performance issues, and you can add as many as you want; for
high-frequency timers (for example, 100 ms), though, every new timer
created makes the UI more sluggish. The preferred solution is to use
only one high-frequency timer.